什么是栈
栈是一种遵从后进先出(LIFO)原则的有序集合。新添加或待删除的元素都保存在栈的同一端,称作栈顶,另一端就叫栈底。在栈里,新元素都靠近栈顶,旧元素都接近栈底。
生活中常见的栈:一摞书、餐厅里叠放的盘子
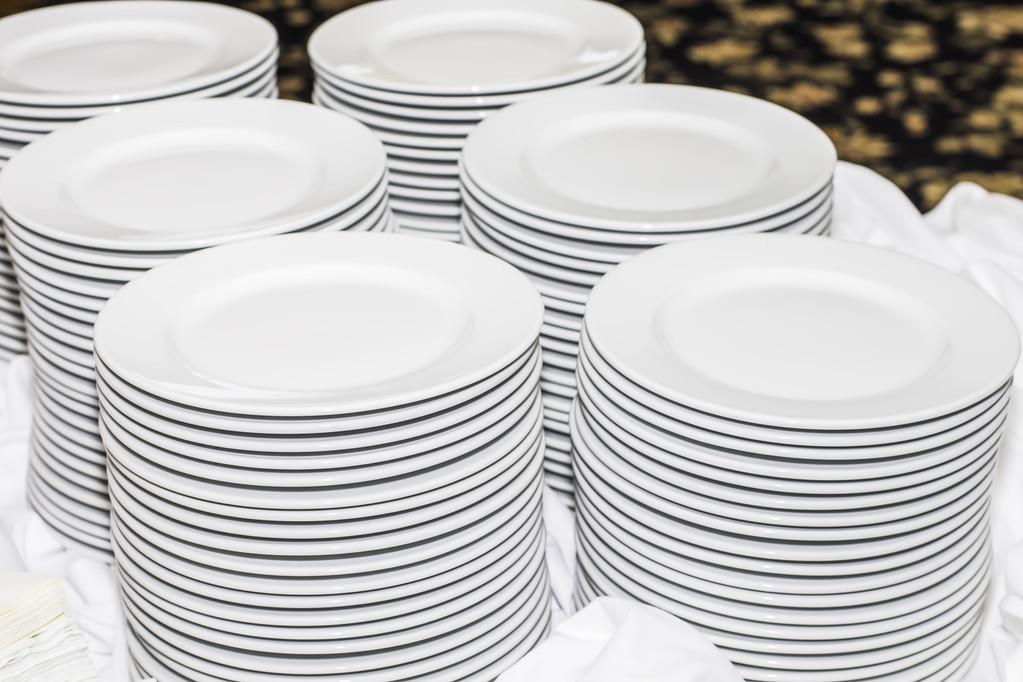
用数组实现栈
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
|
export class Stack { constructor() { this.items = []; }
push(element) { this.items.push(element); }
pop() { return this.items.pop(); }
peek() { return this.items[this.items.length - 1]; }
isEmpty() { return this.items.length === 0; }
clear() { this.items = []; }
size() { return this.items.length; }
toArray() { return this.items; }
toString() { return this.items.toString(); } }
|
用对象实现栈
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83
|
export class Stack { constructor() { this.count = 0; this.items = []; }
push(element) { this.items[this.count] = element; this.count++; }
pop() { if (this.isEmpty()) { return undefined; } this.count--; const result = this.items[this.count]; delete this.items[this.count]; return result; }
peek() { if (this.isEmpty()) { return undefined; } return this.items[this.count - 1]; }
isEmpty() { return this.count === 0; }
clear() { this.items = {}; this.count = 0; }
size() { return this.count; }
toString() { if (this.isEmpty()) { return ''; } let objString = `${this.items[0]}`; for (let i = 1; i < this.count; i++) { objString = `${objString},${this.items[i]}`; } return objString; } }
|